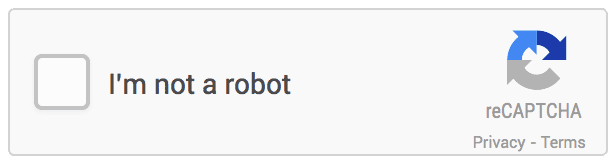
November 2019: Wix has, in the mean time, finally crafted a native (wix) version of the Google reCaptcha. So this contribution can now be regarded as deprecated.
Abstract
This post describes how to add Google´s recaptcha (V2) to a Wix form in Wix Code. We use a Wix html-component to display the recaptcha on a Wix Code form. The recaptcha token that is received in this component is sent back to the main Wix page using postMessage. A backend function does the ultimate verification.
Warning
Please get this example working as is, without adding anything else. Once you get it working, study the code and then integrate it into your own form, so you will at least always have a working example.
1. Preparation
Make a new page in your project (give it any name you wish) and draw a html-component on it. Now paste this script into it:
<!DOCTYPE html>
<html>
<body>
<p>Click the button to display the entire URL of the html-component.</p>
<button onclick="myFunction()">Do it</button>
<p id="demo"></p>
<script>
function myFunction() {
var x = location.href;
document.getElementById("demo").innerHTML = x;
}
</script>
</body>
</html
Now run this script in Preview Mode. It will probably give you a URL that contains
htmlcomponentservice.com
Now Publish this page and run it in your browser. This time it will probably give you a URL like
www-yourdomainname-com.filesusr.com/
The first one is where the html-component is running from in Preview Mode, the second in Publish Mode. That URL that kind of looks like your own domain name is part of Wix´s CDN.
Copy these two URL´s and paste them into Notepad or something. We are going to need them later on.
2. Create your recaptcha keys
Go to http://www.google.com/recaptcha/admin and create your key pair. Choose V2. Google will display two keys, a site key and a secret key. Copy both to Notepad or something.
Now, below the keys there´s a window where you can add the domain names (1 per line) where the above keys will be used from. Here, enter:
your real domain name (e.g. mydomain.com , without the www-part)
the first domain name you prepared in step 1 (e.g. file htmlcomponentservice.com)
the second one from step 1 (likely from filesusr.com )
Do not forget 2 and 3, otherwise the recaptcha will NOT work.
3: Resize html-component
Go back to the page you created in step 1. Resize the html-component to W=500, H=180 pixels. Why? Well, we are going to put the recaptcha in an html-component because Wix does not allow access to the DOM. But when a user clicks the box "I am not a robot", it may happen that Google doesn´t believe him. In that case, it will show a question like "Click all cars" and a bunch of photos to click. All this will be displayed inside the html-component. So if you make it too small, the user will have a hard time clicking the correct images.
4: copy script
Copy the below script into the html-component (so delete the script from step 1), replacing "yoursitekey" with the first key Google gave you in step 2.
At the bottom of the script, you will see
<script src="https://www.google.com/recaptcha/api.js?onload=onloadCallback&render=explicit&hl=es"
async defer>
Change hl=es (I use Spanish) into the language you want to use for the recaptcha (like hl=en for English US. A full list is available here: https://developers.google.com/recaptcha/docs/language
<html>
<head>
<script type="text/javascript">
var verifyCallback = function(response) {
window.parent.postMessage(response, "*");
};
var onloadCallback = function() {
grecaptcha.render('myRecaptcha', {
'sitekey' : 'yoursitekey', //replace this with the site key Google gave you
'callback' : verifyCallback
});
};
</script>
</head>
<body style="font-family: verdana;background-color:white;margin:0;padding:0">
<!-- POSTs back to the page's URL upon submit with a g-recaptcha-response POST parameter. -->
<form action="?" method="POST">
<div id="myRecaptcha"></div>
</form>
//replace hl=es with the language of your choice
<script src="https://www.google.com/recaptcha/api.js?onload=onloadCallback&render=explicit&hl=es"
async defer>
</script>
</body>
</html>
5: copy backend code
A recaptcha works in 3 steps:
display the recaptcha and wait for the user to click "I am not a robot"
a Google check that might be immediate or with a challenge ("Click all the traffic signs", etc). This check hands you a validation token and this token is sent back to your main Wix form using postMessage
a verification of this token using your secret key (another call to a Google API)
The verification is done in a backend function, supplying the Secret Key and the users IP-address. Then, Google´s site verification is called using wix-fetch. The response comes back in JSON format and holds, amongst other things, a key"success" with a boolean value (true|false).
Backend functions are NOT visible to users (unlike front end code), so your secret key is safe here.
In the left pane in Wix, look for "Backend", create a new backend file and call it "recaptcha.jsw".
Now delete all standard code from this backend file and paste the below code. Replace "yoursecretkey" with the second key Google gave you in step 2.
//recaptcha.jsw import { fetch } from 'wix-fetch'; export async function verifyCaptcha(gToken, ipAddr) { let boolSuccess = false; const strSecretKey = "yoursecretkey"; const strUrl = "https://www.google.com/recaptcha/api/siteverify?secret=" + strSecretKey + "&response=" + gToken + "&remoteip=" + ipAddr; const httpResponse = await fetch(strUrl, { method: 'post' }); if (httpResponse.ok) { const json = await httpResponse.json(); boolSuccess = await json.success; } return boolSuccess; }
6. Copy Wix Page code
On your Wix page with the html-component, do the following:
name the html-component "frmReCaptcha"
put a standard button under the component window and name it "btnSubmit". Define an onClick event which must be called "btnSubmit_click"
delete any existing code and copy the below code
import {
verifyCaptcha
}
from 'backend/recaptcha';
let gToken = "";
let strIpAddress = "";
$w.onReady( async function () {
strIpAddress= await chkIpAddress ();
$w("#frmReCaptcha").onMessage((event) => {
if (event) {
gToken = event.data;
}
});
});
export async function btnSubmit_click(event, $w) { let boolOK= await verifyCaptcha(gToken, strIpAddress); if (boolOK) { console.log("Verification result = " + boolOK);
//todo: redirect to page or show box or show a message } } export async function chkIpAddress() { const httpResponse = await fetch('https://extreme-ip-lookup.com/json', { method: 'get' }); if (httpResponse.ok) { const json = await httpResponse.json(); const ipaddress = await json.query; return ipaddress; } }
7. Save and Publish
When you now run this Wix page in your browser, press Ctrl-Shift-i. This will open up Developers Tools. Make sure it is set to display "Console". Here, you will see the result of the verification displayed (the above console.log). Or, you can add a text box to your form and display the same value, whatever you want.
Have fun.
Closing remarks
In the above page code, there is this:
if (boolOK) { console.log("Verification result = " + boolOK);
//todo: redirect to page or show box or show a message }
If this returns TRUE, all is well. Then you might like to save a row to the database, initiate a payment process or send an email. Do NOT make the mistake of putting all that code inside that IF!!! Remember, that code is visible to the end user and it can be changed, thereby making the whole check pointless. Instead, do all these actions in a BACKEND module!
Make sure you read and understand this article: https://support.wix.com/en/article/security-considerations-when-working-with-wix-code
Kommentare